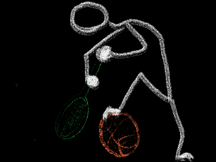
Coding exercises seem like a great idea if you want to learn coding. If you want to learn a practical skill then you should practice, right?
And yet coders don’t do a lot of them. Some, but not a lot. And the longer you’ve been a coder, the fewer of them you seem to do. Ask your favourite long-term veteran coder. A few of them will act guilty that they don’t, but almost none of them actually do coding exercises.
Why don’t we?
I could rail about how silly that is. But I won’t. If nearly everybody doesn’t do something, it’s usually because it’s not as good an idea as it seems. They used to tell us to all use flowcharts for designing program logic. We didn’t. We were 100% right on that one.
We’re basically right about coding exercises, too. But there’s a better alternative that a few people do, especially long-time coders. That’s what you should actually be doing. It’s more fun, too.
But before we get to what you maybe should be doing, let’s talk about why you’re right about most coding exercises.
What Are These Exercises That Don’t Work?
There are many kinds of coding exercises, both common and uncommon. I’ll start with “common” and move toward “more effective.”
Algorithm Challenges
The most common coding exercises are what I call “algorithm of the week” exercises. They describe a simple problem, usually about maths or data structures, and you have to find an effective algorithm to solve it, then code that. Here are some classic examples:
“You have a list where each element links to the next element until the final element (usually) links to nil/null. But if it links back into the same list, it could have a cycle, and so it could be infinitely long. How can you tell if the list I’ve handed you has a cycle or not?” (example solutions)
“Inside every string there is at least one palindrome (words that are the same if you reverse them.) In fact, every individual letter is, technically a palindrome, as is the empty string. But how can you find the length of the longest palindrome inside a given string?” (analysis on Wikipedia)
These are an effective way to practice one very specific subskill of software development: coming up with an algorithm for an unfamiliar data-structures problem. When people criticise these exercises (and that happens a lot,) they criticise them because that subskill isn’t very much of what software developers actually do, day-to-day.
But not only do we not do that very much, it’s often a bad idea to do too much. People who are obsessed with the algorithms with the best asymptotic complexity often ignore the real-world math about the normal size of the data that the algorithm will usually handle. They also tend to ignore a lot of other important factors when treating a real-world application (full of human behaviour) as if it were an oversimplified math problem.
That’s not to say that algorithms problems, asymptotic complexity and analysis are bad. They aren’t. But you have to avoid treating them as the whole problem — they’re just one small aspect of it.
So algorithm challenges like this can be useful, but their usefulness is limited and specific. Once you get good at this skill, you’ll find you don’t need a lot more practice at it.
In their favour, though, these are easy to find. You can join a challenge/exercise site (e.g. TopCoder or exercism.io) and they’ll give you as many of these as you could ever want, immediately and for free. The quality of exercises may vary, of course, and you won’t really know until you’ve worked through them.
Can you “over-practice” these with a bad real-world result? Absolutely. Software shops often tell you to be careful about interview candidates with a high rating on TopCoder or similar challenge sites because they tend to absolutely rock the job interviews, and then do poorly after you hire them. They do poorly because they treat real-world problems like algorithm challenges, and the results don’t work well when you’re trying to solve real problems for real users and get paid to do it. Algorithmic complexity isn’t everything in the real world.
Individual Projects
The far other end from little algorithm challenges is “declare your own project and do it in order to learn.” Just make something up and build it. Or build a tool to “scratch your own itch.” Or try to clone something that somebody else built.
This is a good way to learn to code. Many of the best coders learned this way. But it has some difficulties.
First let’s talk about its good points. You tend to learn a lot, especially if you succeed. If the project is something useful to you, you’ll probably find out whether you did a good job.
There are also down-sides: these projects are often huge, so they take a long time to finish. You may not be making something you want to use (or that ever gets to being usable) and so you may never really find out if you did a good job. If you’re pretty convinced of a bad idea, doing a giant project may reinforce it — you may never realise how you could do better. And it’s so, so easy to pick something gigantic, never finish it, feel guilty that you should be working on it and lose lots of your practice and learning time with very little to show for it.
It’s a huge gamble, basically. You try to build something gigantic, you learn a huge amount if you succeed, and very little if you fail.
Most people find that intimidating, and often don’t want to work on it. So this isn’t everybody’s approach.
Code Katas
Code Katas are a specific kind of coding exercise described by Dave Thomas of Pragmatic Programmers fame.
I don’t meet many people who do them regularly, but they’re great exercises if done well. They don’t even necessarily involve coding (example) and are mostly design exercises. I don’t think coders do enough of that, and I respect how Code Katas present it.
In their favour, they’re about turning an interesting real-world problem into a set of assumptions and algorithmic steps. That is what a coding job involved day-to-day and it’s great practice we don’t get enough of.
Against them, they’re mostly a set of pre-worked examples. It’s not necessarily clear how you would set up your own Code Katas after you finish the 21 of them that Dave put together, or use them to learn deeper skills once you get past his initial choices.
So they’re good exercises. But if you want more or different Code Katas, you’d have to go ask Dave.
There are other, similar exercises. And they have basically the same properties. You can have an excellent teacher (like Dave) put together exercises on a single topic (like OO design) and you’ll get excellent exercises where you still need that teacher to set it up for you. If they don’t teach a topic you want to learn, you’re out of luck.
But on the plus side, you can always use those exercises where you can find them.
Project-Based Books
There are some examples out there of building nontrivial learning projects from a book. There are also series of blog posts that do a similar trick. I’m thinking of books like “99 Bottles of OOP” by Sandi Metz, or Peter Shirley’s “Build a Raytracer in One Weekend.”
They have a lot of good points: the best of them are amazing and you can learn a lot more from a large project than a tiny one-off exercise. If the writer/instructor is good then you can get a lot of feedback on what you’re doing well and poorly. The project is often interesting and it may even be useful when you’re done.
They also have some bad points. First, you’ll need to find them. In my experience the best of these are rare and already famous. The problem isn’t finding 99 Bottles of OOP or The Structure and Interpretation of Computer Programs. The problem is that once you’ve worked through it, what’s next? And since they’re rare, there may not be one that teaches the skill you want. 99 Bottles teaches TDD and Refactoring. But what’s the book for practicing closures or recursion as a real-world project? It’s not clear that there is one, though obviously there are some good books that teach those skills. But if you want a practical exercise to learn to use a given skill in the real world, the book or project you’re looking for may not exist.
Is There a Better Way?
I have the good fortune to be an old-timer at coding. I started coding full-time in 1998 and as a hobbyist in 1984. So I know a lot of old coders and I talk to them a lot. It turns out there are some similarities in what we do… And they look exactly like what a different field does to learn.
Yeah, it turns out that computer programming is slowly, painfully reinventing somebody else’s methods for a fundamental problem. If you’re an old coder, I expect this (*snort*) really shocks you.
It turns out that coders build systems of behaviour. And learning to build things that mimic nature? That’s something humans have been doing for a really long time. And there are some excellent, simple methods of practicing.
I call this adapted exercise a “coding study” — and I have a nice half-hour talk that I gave at RubyConf 2019 about it (see below.)
Who did I steal this exercise from? Artists. Like programmers, they have far too many tools. For artists that means things to draw or paint with. For coders that means languages and libraries. Like programmers, they have a few universal principles to teach that they have to teach through those tools, such as perspective, composition, colour theory and contrast. We have universal principles like modularity, data-hiding and decomposition of tasks which have to be taught via those languages and libraries.
Why Do I Care?
The coding exercises I mention above aren’t bad, and I’m not suggesting you abandon all of them. The problem is that large practical exercises (project-based books, independent projects) are hard to complete, hard to get feedback on, and they’re big gambles — most people don’t complete them and so they don’t get the advantage.
The smaller exercises (Code Katas, algorithms problems) are better about that, but you need to find an excellent coder-slash-teacher to put them together for you (e.g. Sandi Metz, Dave Thomas) and so they probably don’t teach some of the skills you want to learn.
There is absolutely a middle way that fixes these problems. And mostly we don’t do it. If you watch the video above you can learn about coding studies, which:
- don’t require a teacher to set up for you
- don’t take a long time to finish
- are easy to get feedback from
- work great when you’ve just started coding
- work great when you’ve been coding for thirty years
I Hate Videos and/or YouTube
Fair enough. Do you read electronic books? I wrote a book about coding studies. If you sign up for my email list below I’ll send you free chapters from Mastering Software Technique and email you articles about learning coding.
Comments